Sliding window minimum is an interesting algorithm, so I thought I wouldimplement it in a bunch of different languages. This repository contains (orwill contain) implementations of the algorithm in different programminglanguages. What follows is an explanation of the problem and the algorithm.
The repository is stored athttps://bitbucket.org/keegan_csmith/sliding-window-minimum
A copy of this article is stored athttp://people.cs.uct.ac.za/~ksmith/articles/sliding_window_minimum.html
Feb 21, 2019 A Protocol Using Go-Back-N. Go-Back-N protocol, also called Go-Back-N Automatic Repeat reQuest, is a data link layer protocol that uses a sliding window method for reliable and sequential delivery of data frames. It is a case of sliding window protocol having to send window size of N and receiving window size of 1. Selective Repeat Sliding Window Protocol Hilbert's curve Cyclic Redundancy Check Polygon Clipping (Sutherland Hodgman algorithm) Basic transformations(2D):Scaling, Translation, R. Bresenham's circle drawing algorithm Line drawing algorithms How to run C/C graphics programs on Ubuntu. To write a java program to perform sliding window protocol ALGORITHM: 1.Start the program. 2.Get the frame size from the user 3.To create the frame based on the user request. 4.To send frames to server from the client side. 5.If your frames reach the server it will send ACK signal to client otherwise it will send NACK signal to client. 6.Stop the program Program: import java.net.; import java.io.; import java.rmi.; public class slidsender.
The algorithm is sometimes also referred to as the Ascending Minimaalgorithm. I learnt the algorithm from a South African Computer Olympiad campsome years ago. I couldn't find any references to it in any journal, howeverthere are some explanations on the Internet.
Given an array ARR and an integer K, the problem boils down to computing foreach index i: min(ARR[i], ARR[i-1], .., ARR[i-K+1]). The algorithm for this'slides' a 'window' of size K over ARR computing at each step the currentminimum. In other words the algorithm roughly follows this psuedocode:
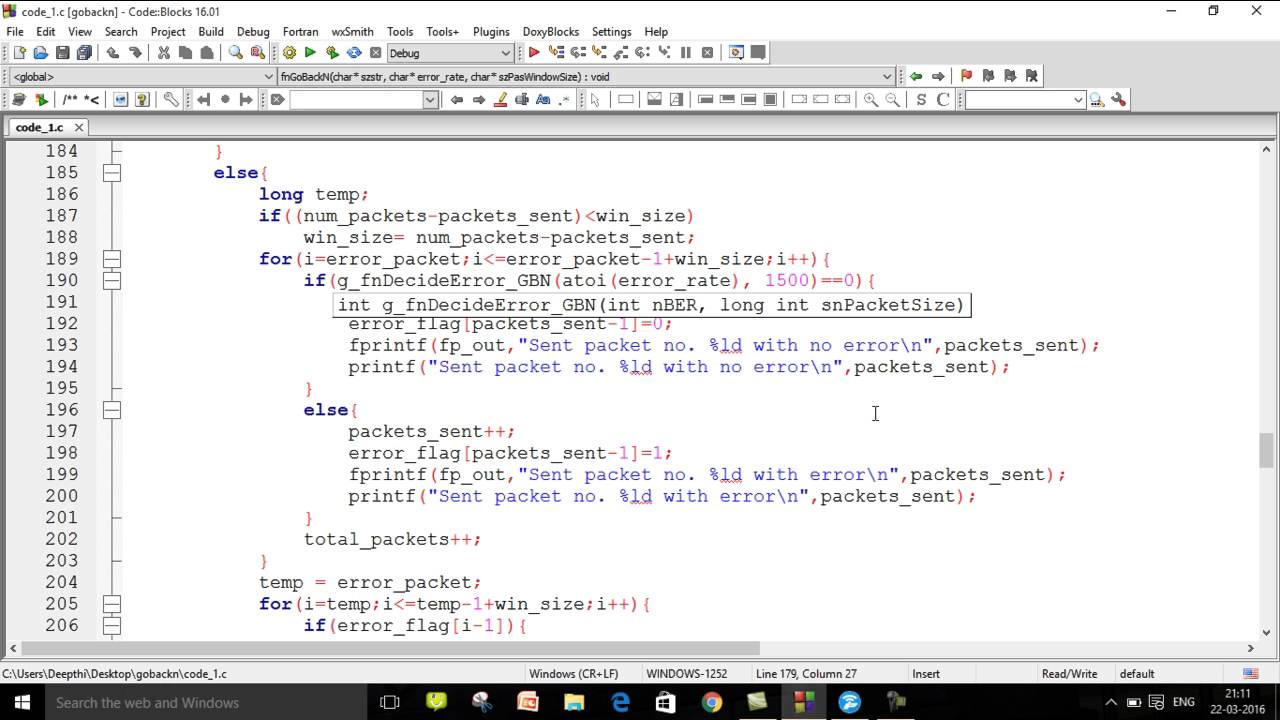
Sample Program In Java Programming
The sliding window algorithm does the remove, insert and output step inamortized constant time. Or rather the time it takes to run the algorithm isO(ARR.size()).
Before I explain the O(1) solution for sliding window minimum, let me explainsome alternative solutions which are suboptimal. The most straight-forwardsolution is for each index i in ARR, simply loop over the values fromARR[i-K+1] to ARR[i] and keep track of the minimum:
The above C++ code runs in O(NK) where N = ARR.size(). We can improve on thisby using a sorted set to speed up the minimum value queries to logarithmictime:
The window can have at most K elements, so the multiset insert, find and beginoperations all take time O(logK). This gives us an overall time of O(NlogK).
We can improve the run-time by a constant factor by using a heap instead. Allthe heap operations (except finding the minima) have the same run-timecomplexity as the multiset versions, however heaps empirically performbetter. We need to be able to remove arbitrary items in the heap, but theimplementation of heaps in C++ (std::priority_queue) does not support thisoperation. Luckily a technique I have mastered in real life helps us getaround this: we delete lazily. For each element in the priority queue we alsostore what index it was inserted from. Then when we query what the smallestelement is, we discard it if its index falls out of range of our currentwindow.:
Note that deleting lazily can actually make this algorithm perform ratherbadly. P90x lean diet plan download. If the input is N values from N to 1, then a value is never deletedfrom the priority queue leading to O(logN) insertions. However, on averagethis is empirically faster than the multiset version.
The idea of lazily deleting elements is a salient one, but by putting in a bitmore effort when inserting an element into the window we can get amortizedO(1) run-time. Say our window contains the elements {1, 6, 7, 2, 4, 2}. Wewant to add the element 5 to our window. Notice that all elements in thewindow greater than 5 will now never be the minimum in the window for future ivalues, so we might as well get rid of them. The trick to this is to store thenumbers in a deque [1] and whenever inserting a number x we remove allnumbers at the back of the deque which are greater than equal to x. Noticethat if the deque was sorted before inserting, it will still be sorted afterinserting x. Since the deque starts off sorted, it remains sorted throughoutthe sliding window algorithm. So the front of the deque will always be thesmallest value.
The front of the queue might have a value which shouldn't be in the windowanymore, but we can use the lazy delete idea with the deques as well. Now eachelement of ARR is inserted into the deque and deleted from the deque at mostonce. This gives as a total run-time of O(N) for the algorithm (amortized O(1)per insertion/deletion). Pretty sweet:
You can modify the algorithm by flipping >= to <= to get the sliding windowmaximum algorithm.
In fact this algorithm works on any totally ordered set. So the elements canbe floats, sets, strings, etc. Essentially anything which has a <= operatorthat behaves 'nicely'.
Think you fully understand this algorithm, try solving these problems:
Learn How To Program In Java
- Task 'sound' at http://www.boi2007.de/en/tasks
- Task 'pyramid' at http://olympiads.win.tue.nl/ioi/ioi2006/contest/day1/(medium to hard problem)
C# Sliding Window Algorithm
[1] | Double-Ended Queue. Supports constant time insertion, removal andlookups at the front and the back of the queue. |